springboot集成thymeleaf
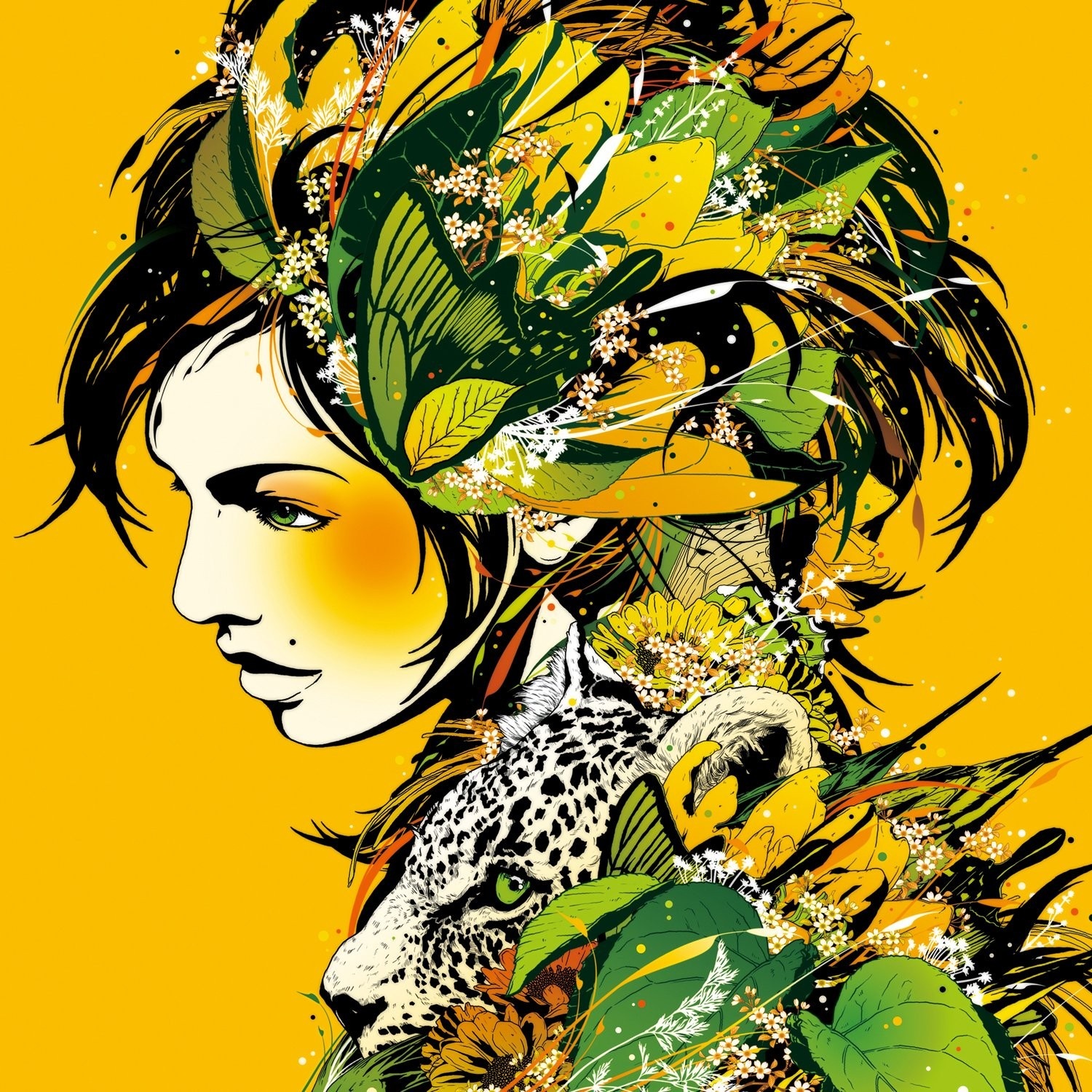
pom.xml
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <parent> <groupId>com.daimeng</groupId> <artifactId>spring-cloud-demo</artifactId> <version>0.0.1-SNAPSHOT</version> </parent> <artifactId>web-thymeleaf</artifactId> <packaging>jar</packaging> <name>web-thymeleaf</name> <description>web-thymeleaf</description> <dependencies> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-thymeleaf</artifactId> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-web</artifactId> </dependency> </dependencies> </project>
application.yml
spring: application: name: web-thymeleaf server: port: 9030 eureka: client: serviceUrl: defaultZone:http://localhost:8555/eureka/ instance: leaseRenewalIntervalInSeconds: 1 leaseExpirationDurationInSeconds: 2
WebServerApplication.java
package com.daimeng.web; import org.springframework.boot.SpringApplication; import org.springframework.boot.autoconfigure.SpringBootApplication; import org.springframework.boot.builder.SpringApplicationBuilder; import org.springframework.boot.web.support.SpringBootServletInitializer; import org.springframework.cloud.client.discovery.EnableDiscoveryClient; @SpringBootApplication @EnableDiscoveryClient public class WebServerApplication { public static void main(String[] args) { SpringApplication.run(WebServerApplication.class, args); } }
WebController.java
package com.daimeng.web.hello.action; import java.util.ArrayList; import java.util.List; import org.springframework.stereotype.Controller; import org.springframework.ui.Model; import org.springframework.web.bind.annotation.PathVariable; import org.springframework.web.bind.annotation.RequestMapping; import com.daimeng.web.hello.data.UserVo; @Controller @RequestMapping("/web") public class WebController { @RequestMapping(value = { "", "/hello/{id}" }) public String hello(@PathVariable Integer id, Model model) { model.addAttribute("muser",new UserVo(id,"张三vo","中国广州vo")); List<UserVo> userList = new ArrayList<UserVo>(); for (int i = 0; i <10; i++) { userList.add(new UserVo(i,"张三"+i,"中国广州"+i)); } model.addAttribute("users", userList); return "/hello/index"; } }
src/main/sources下创建2个目录
static放静态文件,如css/js/img等
templates放html文件
index.html
<!DOCTYPE html> <html xmlns:th="http://www.thymeleaf.org"> <head> <meta charset="UTF-8" /> <title>Insert title here</title> </head> <body> <h2>用户列表-index</h2> <div> <span th:text="${muser.id}"></span> <span th:text="${muser.name}"></span> <span th:text="${muser.add}"></span> <img alt="" src="/img/01.jpg" style="width:50px;height:50px" /> <ul> <li th:each="user:${users}"> <span th:text="${user.id}"></span>- <span th:text="${user.name}"></span>- <span th:text="${user.add}"></span> </li> </ul> </div> </body> </html>
访问效果如图