上传文件
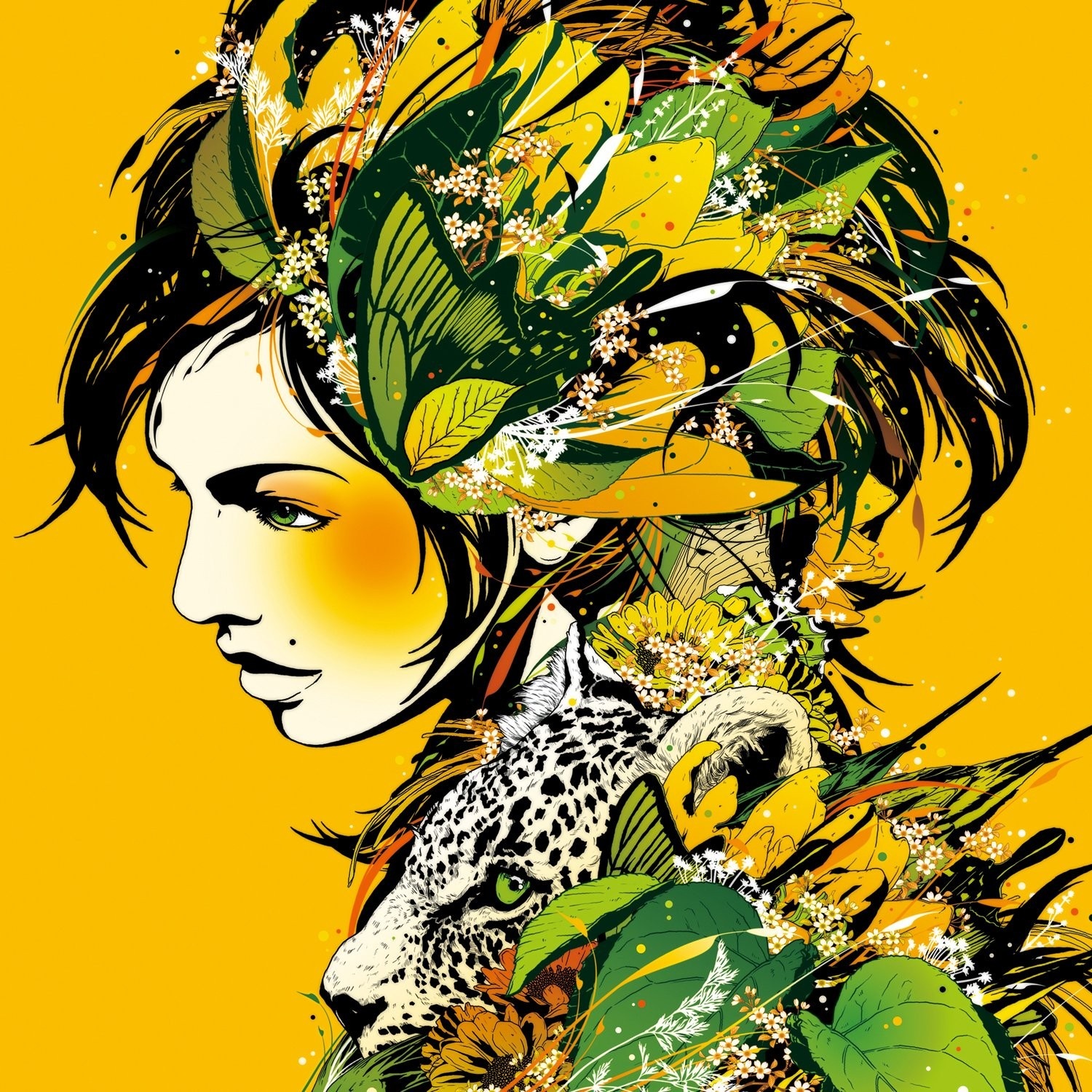
application.yml
spring: #Spring Boot 1.4.x或者之后 http: multipart: maxFileSize: 100Mb maxRequestSize: 1000Mb
FileController.java
package com.daimeng.web.file.action; import java.io.IOException; import java.nio.file.Files; import java.nio.file.Path; import java.nio.file.Paths; import java.util.Map; import org.springframework.stereotype.Controller; import org.springframework.ui.Model; import org.springframework.web.bind.annotation.GetMapping; import org.springframework.web.bind.annotation.PostMapping; import org.springframework.web.bind.annotation.RequestMapping; import org.springframework.web.bind.annotation.RequestParam; import org.springframework.web.multipart.MultipartFile; import org.springframework.web.servlet.mvc.support.RedirectAttributes; @Controller @RequestMapping("/mp") public class FileController { private static String UPLOADED_FOLDER = "D:/java_test/"; @GetMapping("/pg") public String pg(Model model) { return "mapping/upload"; } @GetMapping("/us") public String us(Model model,RedirectAttributes redirectAttributes) { return "mapping/us"; } @PostMapping("/up") //@ResponseBody public String up(@RequestParam("file") MultipartFile file, RedirectAttributes redirectAttributes) { if (file.isEmpty()) { redirectAttributes.addFlashAttribute("message", "请选择一个文件进行上传!"); return "redirect:/mp/up"; } try { // Get the file and save it somewhere byte[] bytes = file.getBytes(); Path path = Paths.get(UPLOADED_FOLDER + file.getOriginalFilename()); Files.write(path, bytes); redirectAttributes.addFlashAttribute("message", "成功上传文件:'" + file.getOriginalFilename() + "'"); } catch (IOException e) { e.printStackTrace(); } return "redirect:/mp/us"; } }
GlobalExceptonHandler.java
package com.daimeng.interceptor; import org.springframework.web.bind.annotation.ControllerAdvice; import org.springframework.web.bind.annotation.ExceptionHandler; import org.springframework.web.multipart.MultipartException; import org.springframework.web.servlet.mvc.support.RedirectAttributes; /** * 设置一个@ControllerAdvice用来监控Multipart上传的文件大小是否受限,当出现此异常时在前端页面给出提示。 * 利用@ControllerAdvice可以做很多东西,比如全局的统一异常处理等 * @author daimeng * */ @ControllerAdvice public class GlobalExceptionHandler { @ExceptionHandler(MultipartException.class) public String handleError1(MultipartException e, RedirectAttributes redirectAttributes) { redirectAttributes.addFlashAttribute("message", e.getCause().getMessage()); return "redirect:/file/uploadStatus"; } }
up.html
<!DOCTYPE html> <html xmlns:th="http://www.thymeleaf.org"> <body> <h1>Spring Boot - 文件上传</h1> <form method="POST" action="/file/upload" enctype="multipart/form-data"> <input type="file" name="file" /><br/><br/> <input type="submit" value="Submit" /> </form> </body> </html>
us.html
<div th:if="${message}"> <h2 th:text="${message}"/> </div>
搜索后评论 搜索后评论 搜索后评论 搜索后评论 搜索后评论