用AST写一个HelloWorld类
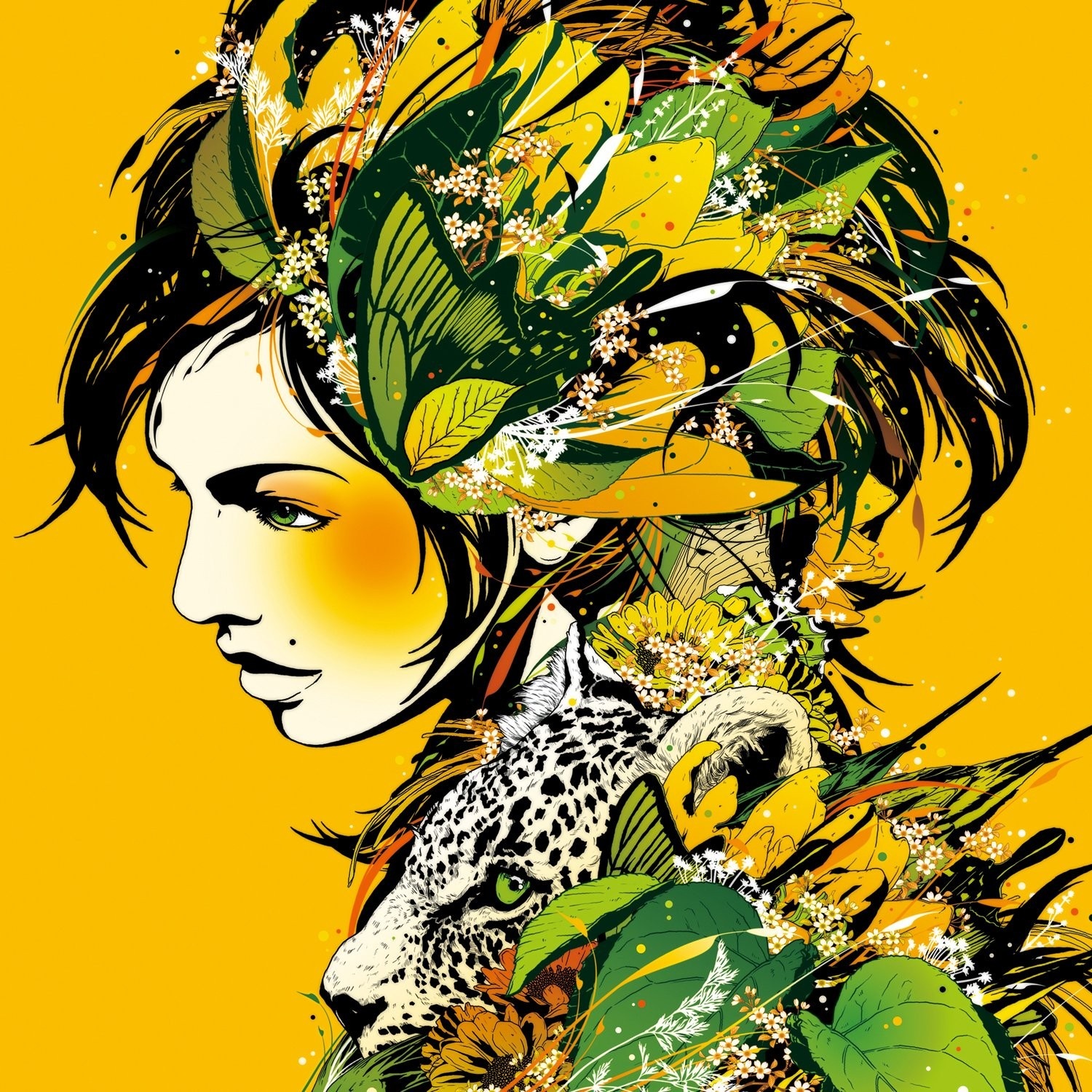
import org.eclipse.jdt.core.dom.*; /** * 用AST写一个HelloWorld类 */ public class HelloWorldBuilder { public static void main(String[] args) { HelloWorldBuilder hb = new HelloWorldBuilder(); } public HelloWorldBuilder() { build(); } private String classNameStr = "HelloWorld"; private void build() { ASTParser parser = ASTParser.newParser(AST.JLS3); parser.setSource("".toCharArray()); CompilationUnit comp = (CompilationUnit) parser.createAST(null); comp.recordModifications(); AST ast = comp.getAST(); /* *public class HelloWorld { * *} */ TypeDeclaration classDec = ast.newTypeDeclaration(); classDec.setInterface(false);//设置为非接口 SimpleName className = ast.newSimpleName(classNameStr); Modifier classModifier = ast.newModifier(Modifier.ModifierKeyword.PUBLIC_KEYWORD); //设置类节点 classDec.setName(className);//类名 classDec.modifiers().add(classModifier);//类可见性 //将类节点连接为编译单元的子节点 comp.types().add(classDec); /* *public class HelloWorld { * public HelloWorld(){ * * } *} */ buildConstructor(ast,classDec); buildMethod1(ast,classDec); //End System.out.println(comp.toString()); } private void buildConstructor(AST ast,TypeDeclaration classDec){ MethodDeclaration methodDec = ast.newMethodDeclaration(); methodDec.setConstructor(true);//设置为构造函数 SimpleName methodName = ast.newSimpleName(classNameStr); Modifier methodModifier = ast.newModifier(Modifier.ModifierKeyword.PUBLIC_KEYWORD); Block methodBody = ast.newBlock(); //设置方法节点 methodDec.setName(methodName);// 方法名 methodDec.modifiers().add(methodModifier);//方法可见性 methodDec.setBody(methodBody); //方法体 //将方法节点连接为类节点的子节点 classDec.bodyDeclarations().add(methodDec); /* *public class HelloWorld { * public HelloWorld(){ * System.out.println("Hello World!"); * } *} */ ExpressionStatement es = buildExpressionStatement4Print(ast,"\"Hello World\""); //将表达式语句ExpressionStatement连接为方法节点的点 methodBody.statements().add(es); } private void buildMethod1(AST ast,TypeDeclaration classDec){ MethodDeclaration methodDec = ast.newMethodDeclaration(); methodDec.setConstructor(false);//设置非构造函数 SimpleName methodName = ast.newSimpleName("doSomething"); Modifier methodModifier = ast.newModifier(Modifier.ModifierKeyword.PRIVATE_KEYWORD); Block methodBody = ast.newBlock(); //设置方法节点 methodDec.setName(methodName);// 方法名 methodDec.modifiers().add(methodModifier);//方法可见性 methodDec.setBody(methodBody); //方法体 //将方法节点连接为类节点的子节点 classDec.bodyDeclarations().add(methodDec); /* *public class HelloWorld { * public HelloWorld(){ * System.out.println("Hello World!"); * } *} */ ExpressionStatement thenE = buildExpressionStatement4Print(ast,"\"Hello World111!\""); ExpressionStatement elseE = buildExpressionStatement4Print(ast,"\"Hello World222!\""); IfStatement is = ast.newIfStatement(); InfixExpression ie = ast.newInfixExpression(); MethodInvocation methodInv = ast.newMethodInvocation(); SimpleName nameSystem = ast.newSimpleName("System"); SimpleName nameTime = ast.newSimpleName("currentTimeMillis"); methodInv.setExpression(nameSystem); methodInv.setName(nameTime); ie.setLeftOperand(methodInv); ie.setOperator(InfixExpression.Operator.GREATER); ie.setRightOperand(ast.newNumberLiteral("0")); is.setExpression(ie); is.setThenStatement(thenE); is.setElseStatement(elseE); //将表达式语句ExpressionStatement连接为方法节点的点 methodBody.statements().add(is); } private ExpressionStatement buildExpressionStatement4Print(AST ast,String str){ MethodInvocation methodInv = ast.newMethodInvocation(); SimpleName nameSystem = ast.newSimpleName("System"); SimpleName nameOut = ast.newSimpleName("out"); SimpleName namePrintln = ast.newSimpleName("println"); //连接‘System’和‘out’ //System.out QualifiedName nameSystemOut = ast.newQualifiedName(nameSystem, nameOut); //连接‘System.out’和‘println’到MethodInvocation节点 //System.out.println() methodInv.setExpression(nameSystemOut); methodInv.setName(namePrintln); //”Hello World!” StringLiteral sHelloworld = ast.newStringLiteral(); sHelloworld.setEscapedValue(str); //System.out.println(“Hello World!”) methodInv.arguments().add(sHelloworld); //将方法调用节点MethodInvocation连接为表达式语句ExpressionStatement的子节点 //System.out.println(“Hello World!”); ExpressionStatement es = ast.newExpressionStatement(methodInv); return es; } }
结果
public class HelloWorld { public HelloWorld(){ System.out.println("Hello World"); } private void doSomething(){ if (System.currentTimeMillis() > 0) System.out.println("Hello World111!"); else System.out.println("Hello World222!"); } }