NACOS学习:01搭建工程
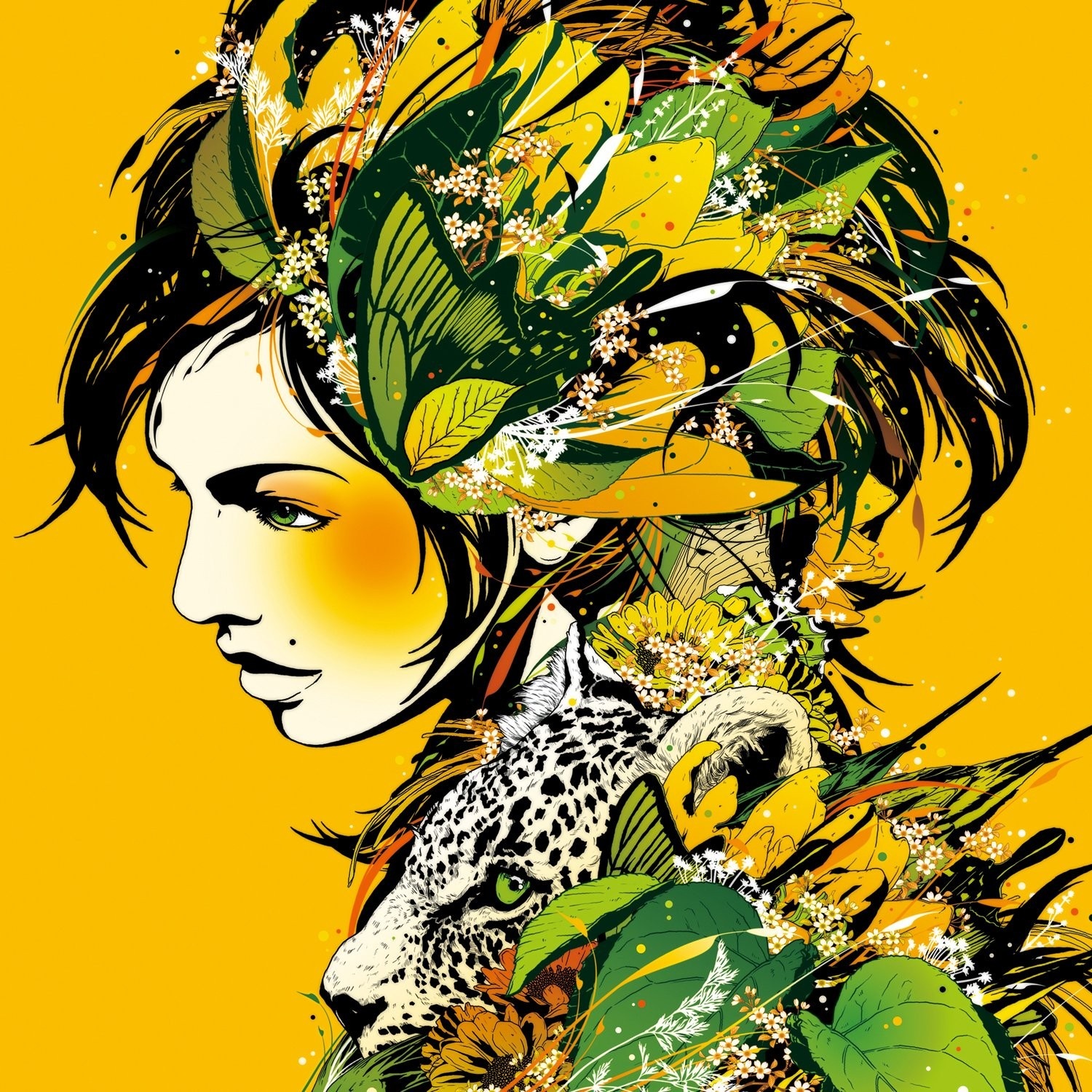
版本概述
spring-boot-starter-parent
<parent> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-parent</artifactId> <!--<version>2.0.4.RELEASE</version>--><!--旧版本,从本版本开始升级的,因为mysql版本6只支持这个--> <!--<version>2.7.3</version>--><!--最新版2022/09/20 只支持JDK17--> <!--<version>2.6.11</version>--><!--支持JDK11--> <version>2.5.14</version><!--支持JDK8--> <relativePath/> <!-- lookup parent from repository --> </parent>
spring-cloud-dependencies
<properties> <project.build.sourceEncoding>UTF-8</project.build.sourceEncoding> <project.reporting.outputEncoding>UTF-8</project.reporting.outputEncoding> <java.version>1.8</java.version> <!--<spring-cloud.version>2021.0.4</spring-cloud.version>--><!--springboot2.6.x和2.7.x对应版本 aka Jubilee--> <spring-cloud.version>2020.0.3</spring-cloud.version><!--springboot2.4.x和2.5.x对应版本 aka Ilford--> <!--<nacos.version>2021.1</nacos.version>--> <nacos.version>2.2.9.RELEASE</nacos.version> </properties> <dependencies> <dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-dependencies</artifactId> <version>${spring-cloud.version}</version> <type>pom</type> <scope>import</scope> </dependency> </dependencies>
微服务模块
pom文件,由原来eureka改为nacos
<dependencies> <dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-starter</artifactId> </dependency> <dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-starter-netflix-eureka-client</artifactId> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-web</artifactId> </dependency> <dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-starter-openfeign</artifactId> </dependency> </dependencies>
nacos
<dependencies> <dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-starter</artifactId> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-web</artifactId> </dependency> <dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-starter-openfeign</artifactId> </dependency> <dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-starter-netflix-hystrix</artifactId> </dependency> <!-- nacos注册中心ribbon与loadbalancer冲突 --> <dependency> <groupId>com.alibaba.cloud</groupId> <artifactId>spring-cloud-starter-alibaba-nacos-discovery</artifactId> <exclusions> <exclusion> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-starter-netflix-ribbon</artifactId> </exclusion> </exclusions> </dependency> <!-- nacos配置中心 --> <dependency> <groupId>com.alibaba.cloud</groupId> <artifactId>spring-cloud-starter-alibaba-nacos-config</artifactId> </dependency> <dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-starter-loadbalancer</artifactId> </dependency> <!--需要使用bootstrap,否则nacos的config不生效--> <dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-starter-bootstrap</artifactId> </dependency> </dependencies>
bootstrap.yml
spring: application: name: spring-cloud-consumer cloud: nacos: username: nacos password: nacos server-addr: http://localhost:8848 discovery: #注册中心地址 server-addr: http://localhost:8848 namespace: df7fd55f-0398-4ceb-97a1-bd240488612c #我们在nacos中创建的空间名称 config: #注册中心地址 server-addr: http://localhost:8848 namespace: df7fd55f-0398-4ceb-97a1-bd240488612c #我们在nacos中创建的空间名称 timeout: 3000 refresh-enabled: true #修改后自动加载 group: DEFAULT_GROUP #nacos配置文件所选择的group prefix: ${spring.application.name} file-extension: yaml #读取nacos配置文件后缀 server: port: 7000 feign: # 开启熔断机制 circuitbreaker: enabled: true # 设置超时时间 httpclient: connection-timeout: 2000
启动类不变
@SpringBootApplication @EnableDiscoveryClient @EnableFeignClients public class ConsumerApplication { public static void main(String[] args) { try { SpringApplication.run(ConsumerApplication.class, args); }catch (Exception e){ // e.printStackTrace(); System.out.println(e.getMessage()); } } }
controller类
import com.sephy.consumer.hello.data.ResponseVo; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.beans.factory.annotation.Value; import org.springframework.cloud.context.config.annotation.RefreshScope; import org.springframework.web.bind.annotation.PathVariable; import org.springframework.web.bind.annotation.RequestMapping; import org.springframework.web.bind.annotation.ResponseBody; import org.springframework.web.bind.annotation.RestController; import com.sephy.consumer.hello.remote.HelloRemote; @RestController @RefreshScope public class HelloController { //NacosConfigProperties //NacosPropertySource //CompositePropertySource @Value("${mengshen.fun.name}") private String name; @Value("${mengshen.fun.address}") private String address; @Value("${mengshen.fun.sex}") private String sex; @Autowired HelloRemote helloRemote; @RequestMapping("/hello/{name}") @ResponseBody public ResponseVo index(@PathVariable("name") String name) { ResponseVo vo = new ResponseVo(); vo.setStatus(100); vo.setDesc(helloRemote.hello(name + " from consumer 1" )); vo.setObj(name+","+address+","+sex); return vo; } }
feign接口类
import org.springframework.cloud.openfeign.FeignClient; import org.springframework.web.bind.annotation.RequestMapping; import org.springframework.web.bind.annotation.RequestParam; @FeignClient(name= "spring-cloud-producer",fallback = HelloRemoteHystrix.class) public interface HelloRemote { @RequestMapping(value = "/hello") public String hello(@RequestParam(value = "name") String name); }
熔断类
import org.springframework.stereotype.Component; import org.springframework.web.bind.annotation.RequestParam; @Component public class HelloRemoteHystrix implements HelloRemote{ @Override public String hello(@RequestParam(value = "name") String name) { return "hello" +name+", this messge send failed "; } }
gateway
pom
<dependencies> <dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-starter-gateway</artifactId> </dependency> <dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-starter-loadbalancer</artifactId> </dependency> <!--<dependency> <groupId>com.alibaba.cloud</groupId> <artifactId>spring-cloud-starter-alibaba-sentinel</artifactId> </dependency>--> <dependency> <groupId>javax.servlet</groupId> <artifactId>javax.servlet-api</artifactId> </dependency> <!-- nacos注册中心 --> <dependency> <groupId>com.alibaba.cloud</groupId> <artifactId>spring-cloud-starter-alibaba-nacos-discovery</artifactId> <exclusions> <exclusion> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-starter-netflix-ribbon</artifactId> </exclusion> </exclusions> </dependency> <!-- nacos配置中心 --> <dependency> <groupId>com.alibaba.cloud</groupId> <artifactId>spring-cloud-starter-alibaba-nacos-config</artifactId> </dependency> <!--需要使用bootstrap,否则nacos的config不生效--> <dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-starter-bootstrap</artifactId> </dependency> <dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-starter-openfeign</artifactId> </dependency> <!--<dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-security</artifactId> </dependency>--> <dependency> <groupId>com.github.penggle</groupId> <artifactId>kaptcha</artifactId> <version>2.3.2</version> </dependency> </dependencies>
bootstrap.yml
spring: application: name: spring-cloud-producer cloud: nacos: username: nacos password: nacos server-addr: http://localhost:8848 discovery: #注册中心地址 server-addr: http://localhost:8848 namespace: df7fd55f-0398-4ceb-97a1-bd240488612c #我们在nacos中创建的空间名称 config: #注册中心地址 server-addr: http://localhost:8848 namespace: df7fd55f-0398-4ceb-97a1-bd240488612c #我们在nacos中创建的空间名称 timeout: 3000 refresh-enabled: true #修改后自动加载 group: DEFAULT_GROUP #nacos配置文件所选择的group prefix: ${spring.application.name} file-extension: yaml #读取nacos配置文件后缀 server: port: 9000
nacos成果
集群
命名空间
服务列表
配置列表
NACOS2:问题集锦